Deal Scenario Management
Create and compare different deal scenarios to find the best overall package, not just the lowest sticker price. Our scenario engine helps you make data-driven decisions.
function compareScenarios(scenarios) {
const results = scenarios.map(scenario => {
const totalCost = calculateTotalCost(scenario);
const monthlyPayment = calculateMonthlyPayment(scenario);
const fiveYearCost = calculateFiveYearCost(scenario);
return {
id: scenario.id,
name: scenario.name,
totalCost,
monthlyPayment,
fiveYearCost,
score: calculateScenarioScore(scenario)
};
});
return results.sort((a, b) => a.score - b.score);
}
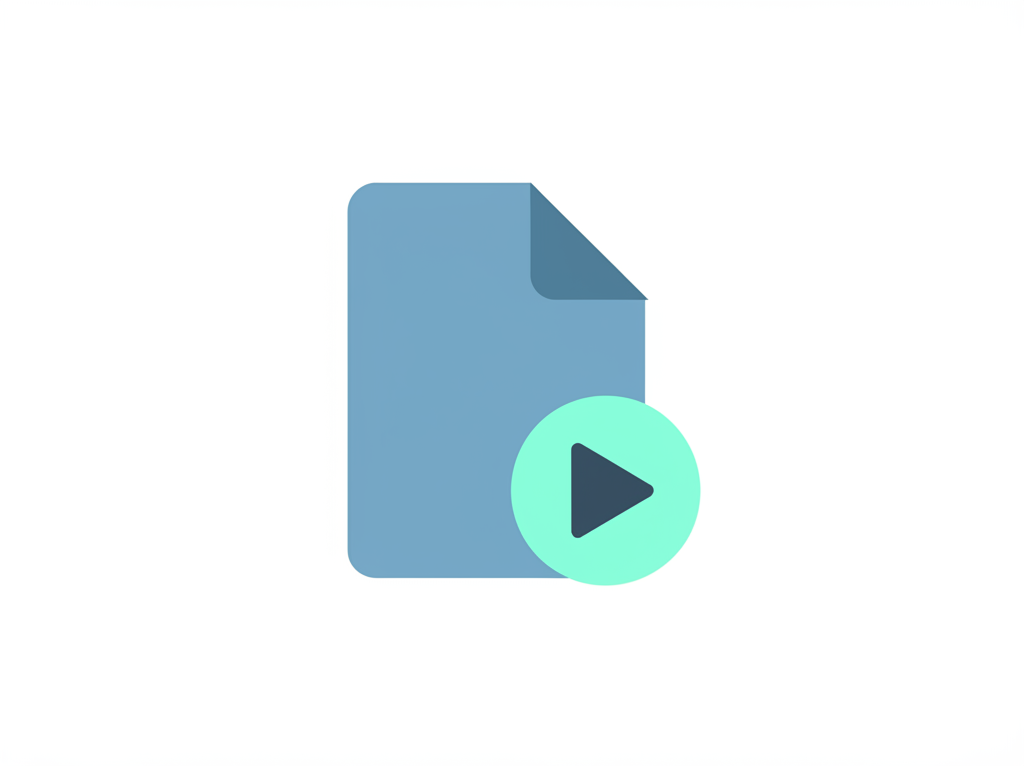
Key Features
Easily compare different deal options side-by-side to see the full picture.
- Total cost of ownership
- Monthly payment breakdown
- 5-year depreciation
Utilize our financial analysis tools to understand the long-term costs of each deal.
- Interest cost visualization
- Tax and fee breakdown
- Equity position tracking
Create and test different scenarios to find the optimal combination of terms.
- Down payment amounts
- Loan term variations
- Interest rate options
Keep all your deal-related documents organized in one place for easy reference.
- Price quotes
- Financing offers
- Trade-in valuations
Interactive Scenario Comparison
See how different scenarios stack up against each other with our interactive comparison tool.
Scenario | Vehicle Price | Down Payment | Trade-In | APR | Term | Monthly | Total Cost |
---|---|---|---|---|---|---|---|
Scenario A | $35,000 | $5,000 | $8,000 | 3.9% | 60 mo | $404 | $37,240 |
Scenario B | $35,000 | $3,000 | $8,000 | 2.9% | 72 mo | $356 | $38,632 |
Scenario C | $33,500 | $4,000 | $7,500 | 4.5% | 60 mo | $410 | $38,100 |
Financial Analysis Tools
Our platform provides a range of financial analysis tools to help you make informed decisions, powered by advanced algorithms and real-time data.
Payment Calculator
Calculate monthly payments based on different loan terms, interest rates, and down payments. See how changes to each variable affect your payment.
Amortization Schedule
Visualize how your payments are applied to principal and interest over time. Understand when you'll reach positive equity.
Trade-in Equity Analyzer
Determine the optimal time to trade in your vehicle based on depreciation curves and remaining loan balance.
function calculateAmortization(principal, rate, term) {
const monthlyRate = rate / 100 / 12;
const monthlyPayment = principal *
(monthlyRate * Math.pow(1 + monthlyRate, term)) /
(Math.pow(1 + monthlyRate, term) - 1);
let balance = principal;
const schedule = [];
for (let month = 1; month <= term; month++) {
const interestPayment = balance * monthlyRate;
const principalPayment = monthlyPayment - interestPayment;
balance -= principalPayment;
schedule.push({
month,
payment: monthlyPayment,
principal: principalPayment,
interest: interestPayment,
balance
});
}
return {
monthlyPayment,
totalInterest: schedule.reduce((sum, month) =>
sum + month.interest, 0),
schedule
};
}
Case Studies
The Challenge
A customer was comparing three different SUVs with various financing options, trade-in values, and dealer incentives. The complexity of the decision was overwhelming.
- 3 different SUV models
- 5 financing options
- 2 trade-in valuations
- Multiple dealer incentives
The Solution
Using our scenario comparison tool, the customer created 8 different scenarios and analyzed the total cost of ownership for each option.
The customer identified a scenario that saved them $4,000 compared to what initially seemed like the best deal based on sticker price alone.
Ready to compare your options?
Start creating scenarios today and find the best overall deal for your next vehicle purchase.